Overview
To enable MQTT based communication for a device, the following steps are required:
- Create an MQTT-based model in the partners portal.
- Register the device using the HTTP-based Register Device API.
- Connect via MQTT using the authorization data returned by the registration API.
Create an MQTT model in the partners portal
To set up a device using MQTT, you must first create a model for your device in the partners portal.
In the "Add Model" dialog, select MQTT under Communication Protocol. This setting must be configured at model creation time and cannot be changed afterwards.
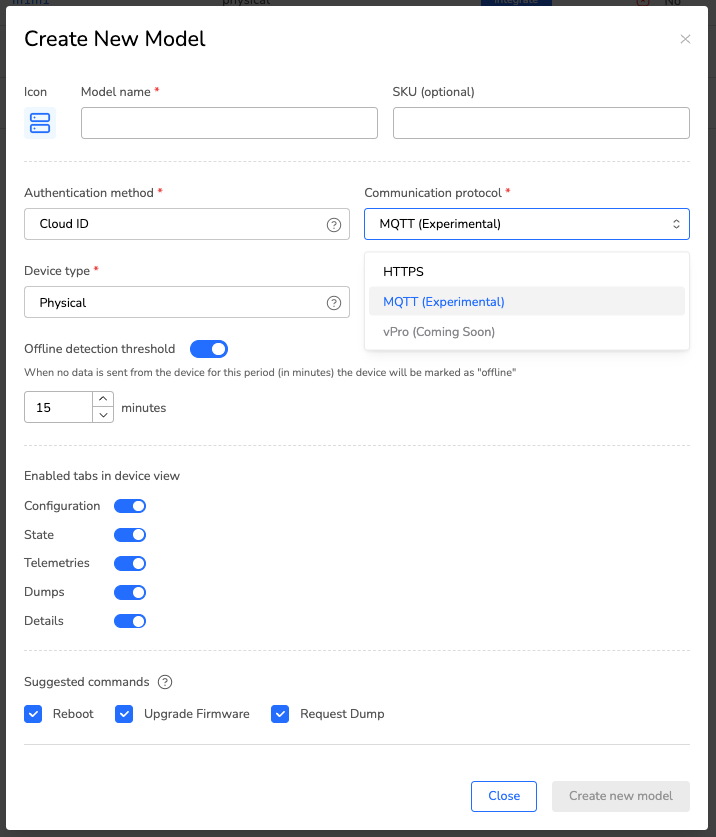
Register a device
Call the HTTPS-based Register Device API. A successful call will return the following parameters, required for establishing MQTT communication:
{
"id": "11111111-2222...",
"access_key": "Secret key for this device...",
"hub_url": "hub-url.com",
"hub_url_static_cert": "hub-url-static.com",
"mqtt_hub_url": "mqtt://mqtt-url.com:port"
}
From this response, you will need three fields:
id
- Unique device IDaccess_key
- Access key used to authenticate with the MQTT servicemqtt_hub_url
- MQTT hub server assigned to this device
The data returned from the Register Device API is shared between the MQTT and HTTPS protocols.
Connect via MQTT
MQTT connection to Xyte requires an MQTT client supporting MQTT v5 for the target programming language.
A comprehensive list of MQTT client implementations can be found at the official MQTT website.
Example of connecting with MQTT.js
To connect, specify the following parameters (from the Register Device API response):
protocolVersion
-5
(this is the MQTT v5 protocol)username
- The unique device IDpassword
- The access key for the deviceclientId
- The unique device ID
MQTT protocol version
Make sure you pass in the protocol version in your chosen client library in order to enable the full features of the v5 protocol.
import mqtt from 'mqtt';
const client = mqtt.connect(URL, {
protocolVersion: 5,
username: DEVICE_ID,
password: ACCESS_KEY,
clientId: DEVICE_ID,
});
Once the client is connected, all subscriptions becomes enabled.